Localization and internationalization
You can build pages for different locales. Plasmic integrates with any localization framework.
The simplest approach to start with is using variants, which we recommend for most users with a small number of locales to support.
Approach 1: use with localization frameworks
If you use something like Lingui, react-intl, or react-i18next, see how to use with localization frameworks.
Approach 2: use variants
You can simply create a global variant group for Locale, adding variants like “en-US”, “de-DE”, etc.
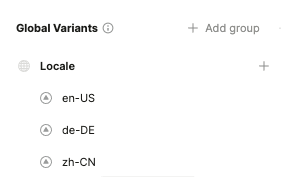
Directly override any content in your pages/components by variant.
Then, render the appropriate variant from your code. This depends on your specific platform’s localization features.
For instance, if you are using Next.js,
you can access your locale information using useRouter
,
then specify the global variant using the React PlasmicRootProvider
API:
// ...export default function MyPage(props: { plasmicData: ComponentRenderData }) {const { locale } = useRouter();return (<PlasmicRootProviderloader={PLASMIC}prefetchedData={props.plasmicData}globalVariants={[{ name: 'locale', value: locale }]}><PlasmicComponent component="MyPage" /></PlasmicRootProvider>);}
Learn more in the official Next.js docs.
Pros:
- Easy to create and integrate into code.
- Easy to maintain consistency across variants.
- Works with components as well as pages.
Cons:
- For hydrating frameworks such as Next.js, the content is part of the bundle, so if you are scaling to many locales, this approach may significantly increase your page weight and is not recommended.
Approach 3: duplicate pages
You can also duplicate your pages, one per desired locale. Then you can just make your desired content changes per locale. For instance, perhaps you adopt a naming convention that ends all your pages with the locale.
Then in code, you fetch and render the appropriate locale:
import L from 'lodash';// ...const { locale } = useRouter();const mungedLocale = L.capitalize(L.camelCase(locale));return <PlasmicComponent component={`${pageName}${mungedLocale}`} />;
Pros:
- Easy to create and integrate into code.
- Adding more locales does not increase page weight.
Cons:
- If you want to keep other changes (layout, etc.) consistent across your locale pages, you will need to manually apply these edits across all the clones.
- Components would need to be forked as well, or use global variants (previous section). You would more likely want to keep most of the content at the page level rather than inside components.
Approach 4: use third-party localization solutions
Services such as LocalizeJS let you specify translations for strings in any rendered page in your app. Plasmic content is no different than other surfaces in your app, so these solutions integrate smoothly with Plasmic.
Have feedback on this page? Let us know on our forum.