Integrating with analytics platforms
External IDs
Plasmic can integrate with third-party analytics platforms using external IDs. When setting up split content, you can optionally assign external IDs to be sent to external platforms.
For each split content, you’ll need to assign the following external IDs:
- Experiment ID, which identifies the specific A/B test, targeted content, or scheduled content
- Variation IDs, one that identifies the original and one that identifies the override
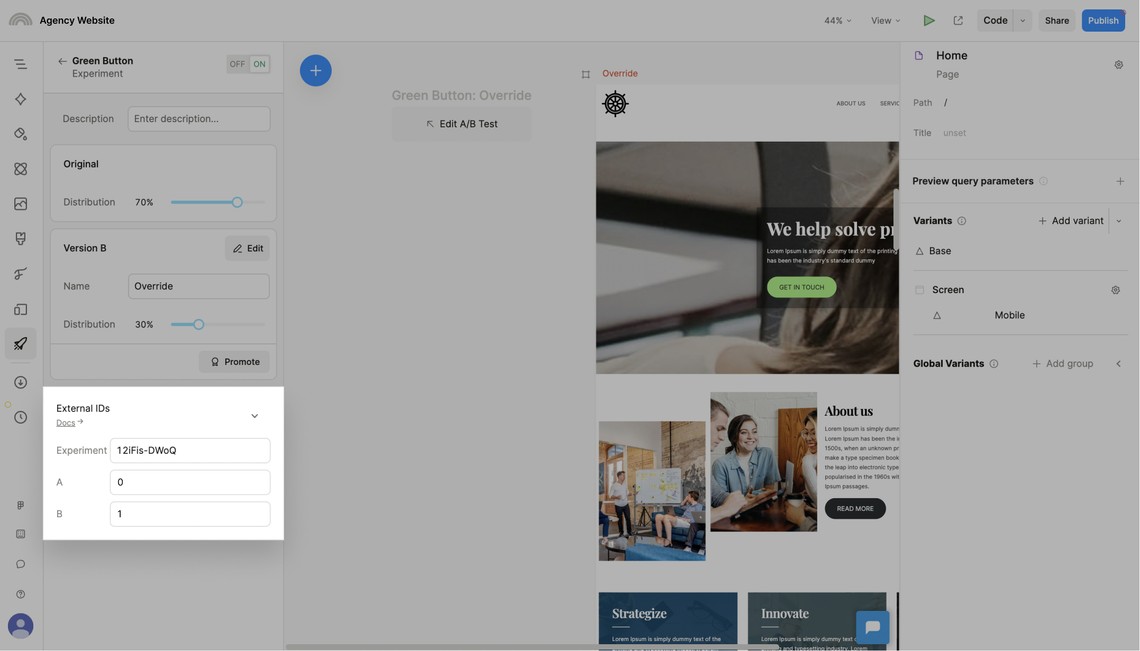
You’ll now need to set up code to send the external IDs to the external platform.
Sending external IDs
Your app is responsible for sending external IDs to the external platform.
In the split content setup, you wrote code for picking the variation to show.
You’ll need to use the variation object to get their external IDs.
getExternalVariation
takes in your variation object and maps them to an object in the form { "EXPERIMENT_ID": "VARIATION_ID" }
.
export const getStaticProps: GetStaticProps = async (context) => {// ...const plasmicData = // ...const variation = // ...const externalIds = PLASMIC.getExternalVariation(variation);return {props: {variation,externalIds,plasmicData,},revalidate: 60,};};
Then, when the page is loaded, you can record the external IDs using your analytics platform’s SDK or API.
Sending external IDs to Google Analytics
Here are instructions to send external IDs to Google Analytics as custom dimensions.
First, create a custom dimension:
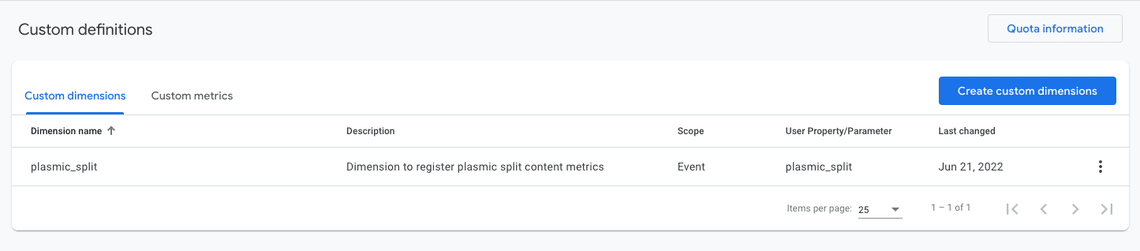
In Plasmic Studio, set the experiment ID to match the custom dimension name. Then, set the variation IDs, which will show up as the values of your custom dimension.
Finally, add some code to send the external IDs on page load. Here’s an example in a Next.js app:
export const getStaticProps: GetStaticProps = async (context) => {// ...const plasmicData = // ...const variation = // ...const externalIds = PLASMIC.getExternalVariation(variation);return {props: {variation,externalIds,plasmicData,},revalidate: 60,};};export default function CatchallPage(props: { plasmicData?: ComponentRenderData; variation?: any, externalIds: any }) {const { variation, externalIds, plasmicData } = props;if (!plasmicData) {return <Error statusCode={404} />;}// Render Plasmic page with the variation picked in `getStaticProps()`return (<>{/* Adds gtag, this can be installed globally, GA_MEASUREMENT_ID is the google analytics ID that you get on your dashboard */}<Scriptsrc={`https://www.googletagmanager.com/gtag/js?id=${GA_MEASUREMENT_ID}`}strategy="afterInteractive"/>{/* Now we only have to append the externalIds as properties to any event that we send to google analytics */}<Script id="google-analytics" strategy="afterInteractive">{`window.dataLayer = window.dataLayer || [];function gtag(){window.dataLayer.push(arguments);}gtag('js', new Date());gtag('config', '${GA_MEASUREMENT_ID}', { 'send_page_view': false });gtag('event', 'pageview', ${JSON.stringify(externalIds)});`}</Script><PlasmicRootProviderloader={PLASMIC}prefetchedData={plasmicData}variation={variation}><PlasmicComponent component={plasmicData.entryCompMetas[0].name} /></PlasmicRootProvider></>);}
In applications composed by multiple pages, getExternalVariation
will return external IDs involving all the projects.
To enable more granular control tracking, getExternalVariation
allows you to filter by project, like this:
export const getStaticProps: GetStaticProps = async (context) => {// ...const plasmicData = //...const variation = // ...const externalIds = PLASMIC.getExternalVariation(variation, {// Only record external IDs used on this page.projectIds: plasmicData.entryCompMetas.map((m) => m.projectId),});return {props: {variation,externalIds,plasmicData,},revalidate: 60,};};
You can see a complete working example of this approach.
Setting external IDs from an experimentation platform
See an example repo demonstrating A/B testing that is synced with split.io in a Next.js.
Many experimentation platforms include functionality for choosing the variation. In this case, we can also use external IDs to set the variation.
In Plasmic Studio, assign the experiment IDs and variation IDs to match the IDs in your experimentation platform.
Then, in the variation
prop of PlasmicRootProvider
, add a key in the format "ext.EXPERIMENT_ID"
with the variation ID as the value.
Here’s an example using @marvelapp/react-ab-testing
:
<Experiment name="My Example"><Variant name="A"><PlasmicRootProvider loader={PLASMIC} variation={{ 'ext.My Example': 'A' }}>{/* YOUR PLASMIC COMPONENTS HERE */}</PlasmicRootProvider></Variant><Variant name="B"><PlasmicRootProvider loader={PLASMIC} variation={{ 'ext.My Example': 'B' }}>{/* YOUR PLASMIC COMPONENTS HERE */}</PlasmicRootProvider></Variant></Experiment>
Have feedback on this page? Let us know on our forum.