Dynamic values
When you add a text element and type in some text content like “Jane Doe,” you’re setting that text to a fixed or static value.
However, let’s say you want that text to show the name of the current user. Or you want it to show the description of the current product that the user is looking at. There needs to be a way for the user to set the text to “whatever is the current user’s name,” or “whatever is the product currently being viewed.”
Then you want that text to show a dynamic value.
Dynamic values are used all over the place in Plasmic Studio:
- Connect element properties (e.g. text content, props, visibility, and variants) to data to build interactive user experiences.
- Work with interactions and state
- Optionally, transform or process data with code expressions
Dynamic values can use any data available in the project, such as from data queries, state variables, and data provided from code components.
Using dynamic values
You can usually set a dynamic value by right-clicking a property and clicking “Use dynamic value”. This will bring up the data picker, which shows you all the data available in the current component or page.
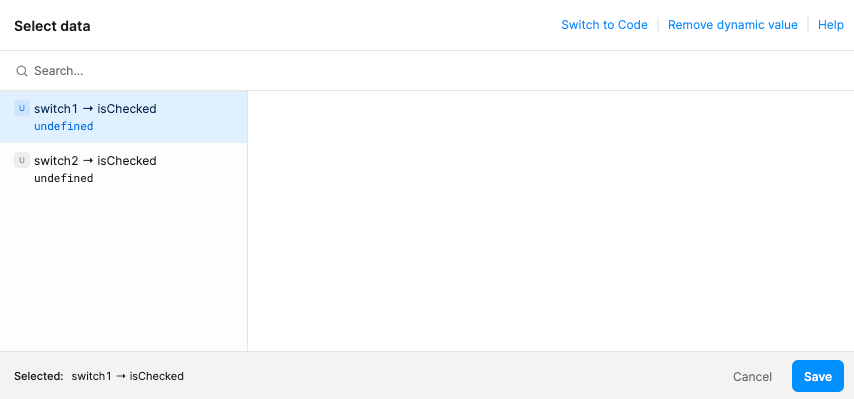
Fallback value
A fallback value is used if the dynamic value includes a data query that is still loading.
If you are unexpectedly seeing the fallback value, there might be an error evaluating the dynamic value.
A common error is when you try to access an object that is null
or undefined
.
You can try to fix this by using optional chaining or conditional expressions.
Missing a data query?
Data queries can only be used from the same component or page. Read the data queries documentation to learn how to fix this issue.
Missing a state variable?
If you’re not seeing a state variable from your own stateful component, you might need to configure its external access.
Video examples
Tutorial video
Connect text to dynamic data
Connect props to dynamic data
Beyond content, bind any attribute/prop to data, such as image sources, link destinations, and custom props:
Make elements repeat according to data
Conditionally show/hide elements
Use JavaScript code expressions in dynamic data
Writing code expressions
Writing code is optional. You can build many interactive experiences without writing a single line of code. However, adding just a sprinkling of code can take your projects to the next level.
If you want to transform or process data, click “Switch to Code” to open the code editor. The currently selected data will automatically be converted to code for you.
Code expressions are JavaScript code with a few limitations.
Common code expressions
Type | Purpose | Code expression |
---|---|---|
Boolean | Invert a boolean | !$state.boolean |
Boolean | Check a AND b | $state.a && $state.b |
Boolean | Check a OR b | $state.a || $state.b |
Boolean | Invert a boolean | !$state.boolean |
Text | Get length of text | $state.text.length |
Text | Check text is not empty | $state.text.length > 0 |
Text | Concatenate text | "Hello, " + $state.givenName + " " + $state.familyName |
Text | Substitute text | `Hello, ${$state.givenName} ${$state.familyName}` |
Number | Compare a number | $state.number > 0 $state.number <= 10 |
Number | Round a number | Math.round($state.number) |
Number | Round a number down | Math.floor($state.number) |
Number | Round a number up | Math.ceil($state.number) |
Number | Add numbers | $state.a + $state.b |
Number | Subtract numbers | $state.a - $state.b |
Number | Multiply numbers | $state.a * $state.b |
Number | Divide numbers | $state.a / $state.b |
Array | Get length of array | $state.array.length |
Array | Access item in array | $state.array[i] |
Object | Access value in object by name Note arrays are 0-indexed, so the first item is 0 | $state.object["name"] $state.object.name |
Object | Optional chaining Access only if object exists | $state.object?.name |
General | Conditional expression | $state.boolean ? $state.text : "Fallback text" |
Special global variables
Data is referenced via a few special global variables:
Global variable | Description |
---|---|
$state | State variables available to the current component or page. |
$props | Props passed into the current component. |
$ctx.pagePath | Page URL path, with path parameters filled (e.g. /products/123 ). |
$ctx.pageRoute | Page URL path (e.g. /products/[id] ). |
$ctx.params | Page URL path params (e.g. /path/[path_param]/ ). |
$ctx.query | Page URL query params (e.g. /path?query_param=<value> ). |
$ctx.<name> | Any other data provided by code components. |
$queries | Object containing data from data queries. Link to documentation |
$steps | Object containing data from previous actions. Only available in interactions. Link to documentation |
currentUser | Object containing data about the logged-in user. Only available when auth is enabled. Link to documentation |
Limitations
Using window
object
In most cases, the best practice is to avoid accessing variables within the window
object. Instead, rely on page metadata stored in context or other designated storage locations. Accessing window
directly can lead to hydration errors that are difficult to resolve and may cause unexpected results during the SSR (Server-Side Rendering) phase.
If you’re certain there’s no alternative, access window via globalThis.window
and use optional chaining for added safety.
Available global properties
Only the following properties are permitted to be used within expressions:
Array
Boolean
Date
Infinity
Intl
JSON
Map
Math
NaN
Number
Object
ReferenceError
RegExp
Set
String
TypeError
clearInterval
clearTimeout
console
localStorage
sessionStorage
setInterval
setTimeout
undefined
globalThis.window
Have feedback on this page? Let us know on our forum.